In this blog post, you will learn what data coupling is and why it’s important. Also, you will see a pythonic code sample that exemplifies the concept. This blog post is part 1 of a series on pythonic coupling.
What Is Data Coupling and Why Is It Important?
Data coupling is when two or more modules are coupled by sharing primitive data types (Ingeno, 2018). Ideally, the modules are completely unaware of the other module’s existence in the sense that they do not directly invoke each other.
The reason data coupling is important is because it loosely couples the modules, and in fact, it is the loosest type of coupling you can have except for no coupling, which means that the modules are completely independent and they do not even pass data to one another (Samir, 2023). Therefore, data coupling is highly preferred over other types of tighter coupling, such as stamp coupling.
A Pythonic Data Coupling Example
The following pythonic example will demonstrate a data coupling between a product module, taxes module, and receipt module. The code is a simple example of purchasing a product, which involves calculating the subtotal, tax total, total, and displaying a receipt of the purchased product.
The following is the code of the product.py module:
class Product:
def __init__(self, name : str, cost : float):
self.__name = name
self.__cost = cost
@property
def name(self) -> str:
return self.__name
@property
def cost(self) -> float:
return self.__cost
The following is the code for the taxes.py module:
def compute_sales_tax(SALES_TAX : float, amount : float):
return round(amount * SALES_TAX, 2)
The following is the code for the receipt.py module:
def __print_separator():
print("------------------------------------------------------------------------")
def display_receipt(product_name : str, cost : float, subtotal : float, total_tax : float, total : float):
__print_separator()
print("Receipt")
__print_separator()
print("Products\n")
print(product_name, "\t\t", "$", cost)
__print_separator()
print("\t\t\t\t\t", "Subtotal: ", "\t $", subtotal)
print("\t\t\t\t\t", "Total Tax: ", "\t $", total_tax)
print("\t\t\t\t\t", "Total: ", "\t $", total)
__print_separator()
The following is the code for main.py, which demonstrates how these modules interact:
from product import Product
import taxes
import receipt
def main():
# The taxes module, receipt module, and product module are all blissfully unaware
# each other. They are only coupled by the data returned from the modules, and
# passed into the next module.
# Visit the following URL to purchase the mug :)
# URL: https://bestsoftwareengineer.com/product/ceramic-mug-11oz/
product : Product = Product("Best Software Engineer Ceramic Mug 11oz", 7.33)
SALES_TAX = 0.06
# Calculate the subtotal, total_tax, and total.
subtotal : float = product.cost
total_tax : float = taxes.compute_sales_tax(SALES_TAX, subtotal)
total : float = round(subtotal + total_tax, 2)
# Display the receipt.
receipt.display_receipt(product.name, product.cost, subtotal, total_tax, total)
if __name__ == "__main__":
main()
You will notice in the code above that the product module, taxes module, and receipt modules are all unaware of each other, and only primitive data types are shared between the modules. For example, the cost of the product from the product module is passed to the compute_sales_tax function of the taxes module, and the product name, product cost, and total tax is shared with the receipt module from the product module and taxes module.
The following is the output when running main.py:
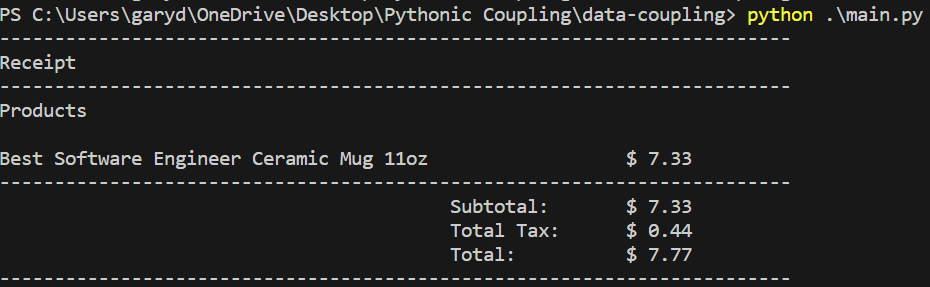
That wraps up this blog post on pythonic data coupling. You learned what data coupling is and why it’s important, and you were given a code sample that exemplifies data coupling in python.
If you found this blog post helpful, then you should share it, subscribe to my blog, and consider buying me a coffee, so I can keep producing these blog posts and digital content for you.
References
Ingeno, J. (2018). Software architect’s handbook. Packt Publishing.
Samir, A. (2023, August 18). Data Coupling – Types Of Coupling. Linkedin.com. https://www.linkedin.com/pulse/data-coupling-types-ofcoupling-ahmed-samir-ahmed